PHP Training
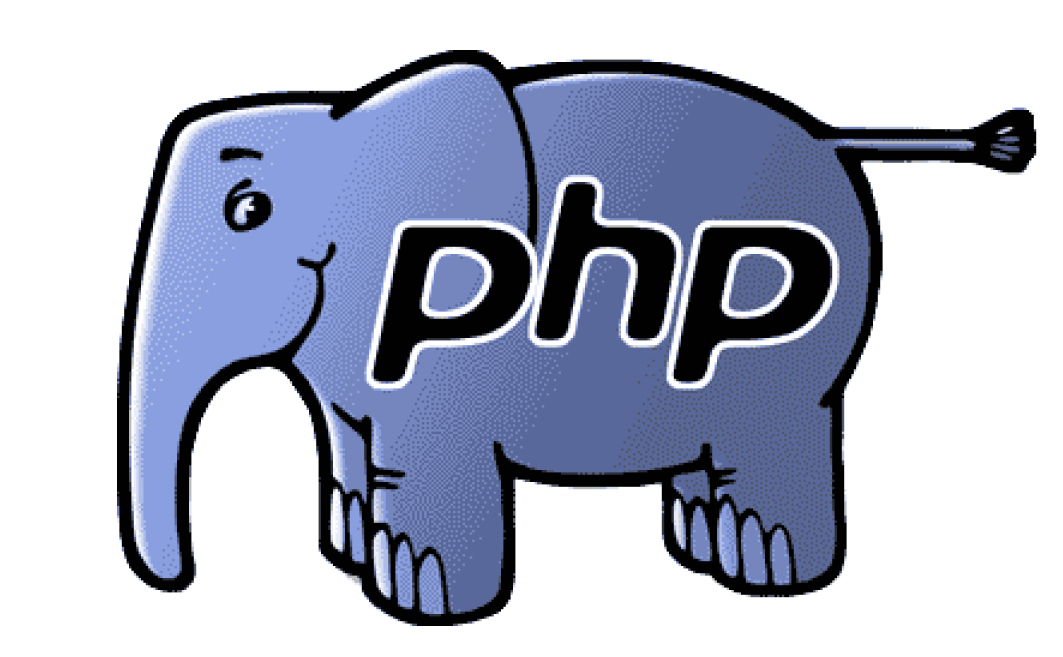
PHP
Learn the PHP language to be able to make your application interact with a database
French / English
Certificate
Overview
Add a registration and login interface
– Allow new users to register and login
– Store passwords securely in the database
– Restrict content to logged-in users only
– Use sessions to manage logins
– Use cookies to possibly remember the user’s login
– Allow users to securely reset their own passwords
Create registration and login using templates, views and controllers
Most websites and web applications require user authentication. Once users have registered on your site, you can customize content specifically for them and restrict content to logged-in users only.
By developing user authentication using MVC architecture, your code will be easier to write and maintain.
All the concepts you need to know to understand why the system is developed the way it is are explained in detail.
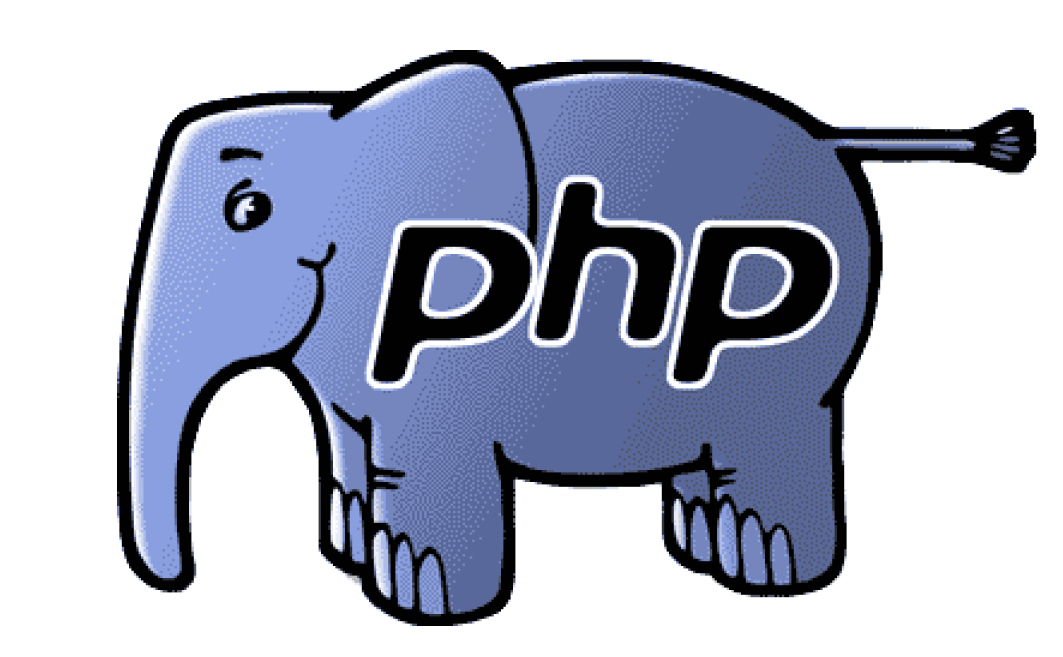
Prerequisite
You should already be familiar with the basics of PHP, HTML, CSS and JavaScript
You should be comfortable installing software on your computer
You should be familiar with the model - view - controller (MVC) model
Goals
Allow new users to create a new account
Validate user registration data, both on the server and in the browser
Store passwords securely in the database
Allow users to log in using their email and password
Restrict content to logged-in users only
Use sessions to manage logins
Use cookies to possibly remember the user's login
Allow users to securely reset their own passwords when they forget them
Allow users to update their own profile details
Training Program
PRESENTATION AND SET-UP OF THE PROJECT
- Installation of the PHP server and the database server on the machine
- Creating a new project and architecting the project according to the MVC model
- Creation of a new database
NEW USER REGISTRATION
- Generate password hashes
- Create a table in the database to store user accounts
- Create and display the registration page
- Add a form to the registration page
- Create the registration action in the controller
VALIDATE THE REGISTRATION DATA IN THE BROWSER
- Validate the registration page in the browser using HTML5 validation
- Add a JavaScript validation library
- Validate the registration page in the browser using JavaScript
- Validate the password format with a custom validation method
- Validate that the email address is unique using an Ajax request
- The password confirmation field: why it's a problem and how to fix it
- Remove the password confirmation field and add a Show Password button
- Validate registration data in the browser
VALIDATE THE REGISTRATION DATA ON THE SERVER
- Validate that the email address is unique in the user table
- Display validation error messages in the registration form
- Prevent duplicate form submissions by using the Published / Redirect / Get template
- Redirect to another page in PHP: how, why and best practices
- Redirect to the success page after a successful registration
- Validating registration data on the server
LOGIN : AUTHENTICATE USER WITH EMAIL ADDRESS AND PASSWORD
- Create the login action in the controller
- Find the user object using the email address
- Authenticate the user by verifying that their password is correct
- Re-display the email address in the login form when authentication fails
- Add a redirection method to the main controller
- Sessions in PHP: make the web browser remember you
- Use session to remember the connection and display the connection status
- Sessions in PHP: completely destroy a session, even without closing the browser
- Destroy the session to disconnect the user
- Sessions in PHP: prevent session fixation attacks
- Login
RESTRICT ACCESS TO AUTHENTICATED USERS ONLY
- Restrict a page to logged in users only
- Redirect to the originally requested page after login
- Add a method to the main controller to require login
- Require login for all action methods in a controller
- Add a base controller that requires login for all action methods
- Get the current authenticated user in controllers and views
- Simplify the code: remove the isLoggedIn method
FLASH MESSAGE : DISPLAY STATUS MESSAGES TO USERS
- Add a flash message on login request
- Display flash messages to user
- Add flash message on login
- Add flash message on logout
- Add CSS and style flash messages
- Add flash message types and style them differently
REMEMBER ME : GIVE USERS THE ABILITY TO REMEMBER LOGIN CREDENTIALS
- Generate unique random tokens and secure hashes
- Add a class to generate and create random token hashes
- Create a database table to store remembered connections
- Add a Remember me checkbox to the login form
- Store login in database
- Cookies in PHP: the basics
- Remember login in a cookie
- Automatically login using the token in the cookie
- Prevent automatic login if the storage token has expired in the database
- Forget the stored login when logging out
- Remember me
PASSWORD RESET PART 1 : SECURELY REQUEST A FORGOTTEN PASSWORD RESET
- Access an email service
- Add a class to send emails
- Create and display the forgotten password page
- Process the forgotten password form in the controller
- Add password reset fields to user table in database
- Register new password reset token and its expiration with user registration
- Send the password reset email to the user
- Get the content of the email from a view template
PASSWORD RESET PART 2 : SAFELY RESET A FORGOTTEN PASSWORD
- Get the user based on the token and check the expiration
- Create and display the password reset form
- Extract the repeated JavaScript code into a separate file
- Process the password reset form in the controller
- Remove the duplicate code and add an expired token view
- Validate the password reset form on the server
- Reset the user's password and clear the token and expiration
- Reset password